10 Advanced Python Concepts That AI Knows but Maybe Not You
Python is a versatile language, but to truly stand out as an advanced Python developer, you must master deeper and more powerful concepts. This blog post explores 10 advanced Python topics every serious Pythonista should understand, complete with examples.
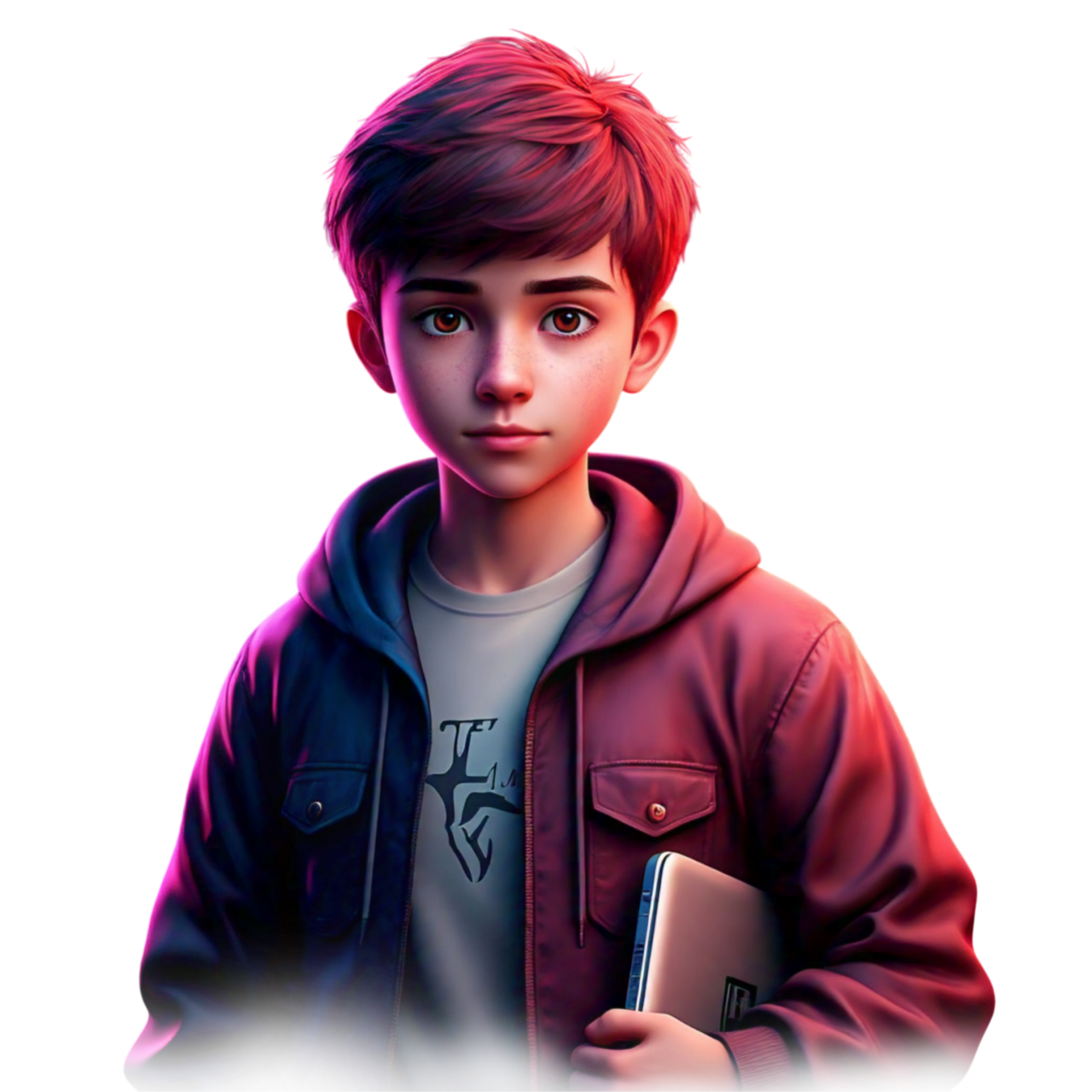
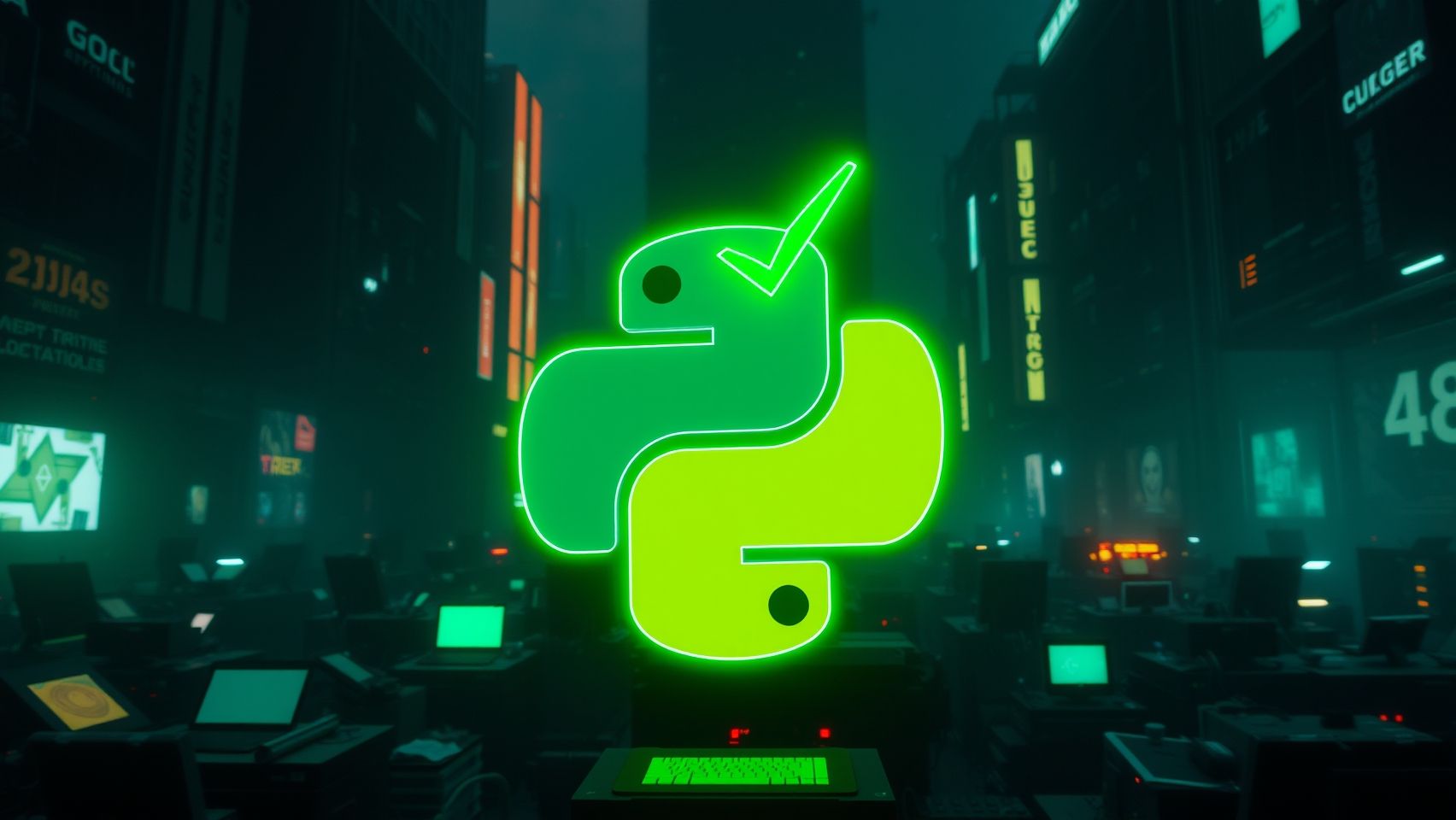
10 Advanced Python Concepts That AI Knows but Maybe Not You
1. Metaclasses
Metaclasses allow you to control class creation, making them a cornerstone of Python’s flexibility.
class Meta(type):
def __new__(cls, name, bases, dct):
print(f"Creating class {name}")
return super().__new__(cls, name, bases, dct)
class MyClass(metaclass=Meta):
pass
Key Takeaway:
Master metaclasses to customize how classes behave and enable powerful design patterns.
2. Descriptors
Descriptors manage attribute access in a class.
class Descriptor:
def __get__(self, instance, owner):
return instance._value
def __set__(self, instance, value):
instance._value = value
class MyClass:
attr = Descriptor()
obj = MyClass()
obj.attr = 42
print(obj.attr)
Key Takeaway:
Understand how descriptors work to manage and control attribute access effectively.
3. Context Managers
Context managers ensure resources are properly managed.
class MyContext:
def __enter__(self):
print("Entering context")
return self
def __exit__(self, exc_type, exc_value, traceback):
print("Exiting context")
with MyContext() as ctx:
print("Inside context")
Key Takeaway:
Learn to create custom context managers using __enter__ and __exit__.
4. Decorators
Advanced decorators enhance your functions and classes dynamically.
class Meta(type):
def cache(func):
memory = {}
def wrapper(*args):
if args not in memory:
memory[args] = func(*args)
return memory[args]
return wrapper
@cache
def fibonacci(n):
if n <= 1:
return n
return fibonacci(n-1) + fibonacci(n-2)
print(fibonacci(10))
Key Takeaway:
Leverage advanced decorators to optimize and extend functionality.
5. Iterators and Generators
Understand custom iterators and generators for efficient data handling.
class MyIterator:
def __init__(self, n):
self.n = n
self.current = 0
def __iter__(self):
return self
def __next__(self):
if self.current < self.n:
self.current += 1
return self.current
raise StopIteration
for val in MyIterator(5):
print(val)
Key Takeaway:
Master __iter__, __next__, and generator functions for custom iteration logic.
6. Async Programming with asyncio
Take concurrency to the next level using asynchronous programming.
import asyncio
async def task(name):
print(f"Task {name} started")
await asyncio.sleep(1)
print(f"Task {name} finished")
async def main():
await asyncio.gather(task("A"), task("B"), task("C"))
asyncio.run(main())
Key Takeaway:
Deeply understand async, await, and asyncio for high-performance I/O operations.
7. Type Hints and Annotations
Type hints improve code readability and debugging.
from typing import List
def add_numbers(nums: List[int]) -> int:
return sum(nums)
print(add_numbers([1, 2, 3]))
Key Takeaway:
Adopt type annotations to write clean and maintainable code.
8. Slots
Use __slots__ to optimize memory usage in your classes.
class MyClass:
__slots__ = ['attr1', 'attr2']
def __init__(self):
self.attr1 = 0
self.attr2 = 0
obj = MyClass()
obj.attr1 = 42
Key Takeaway:
Use __slots__ to control attribute creation and reduce memory overhead.
9. Multithreading and Multiprocessing
Handle CPU-bound and I/O-bound tasks efficiently.
from multiprocessing import Process
def worker():
print("Worker process")
if __name__ == "__main__":
p = Process(target=worker)
p.start()
p.join()
Key Takeaway:
Learn when to use threads versus processes to maximize performance.
10. C Extensions with Cython
Boost Python’s performance by writing C extensions.
# Example setup.py
from setuptools import setup
from Cython.Build import cythonize
setup(
ext_modules=cythonize("example.pyx")
)
Key Takeaway:
Use Cython to compile Python code into C for significant speed improvements.
Conclusion
Mastering these advanced Python concepts will take your skills to the next level, enabling you to write more efficient, maintainable, and powerful programs. Embrace these techniques to truly call yourself an advanced Python developer.
About the Author
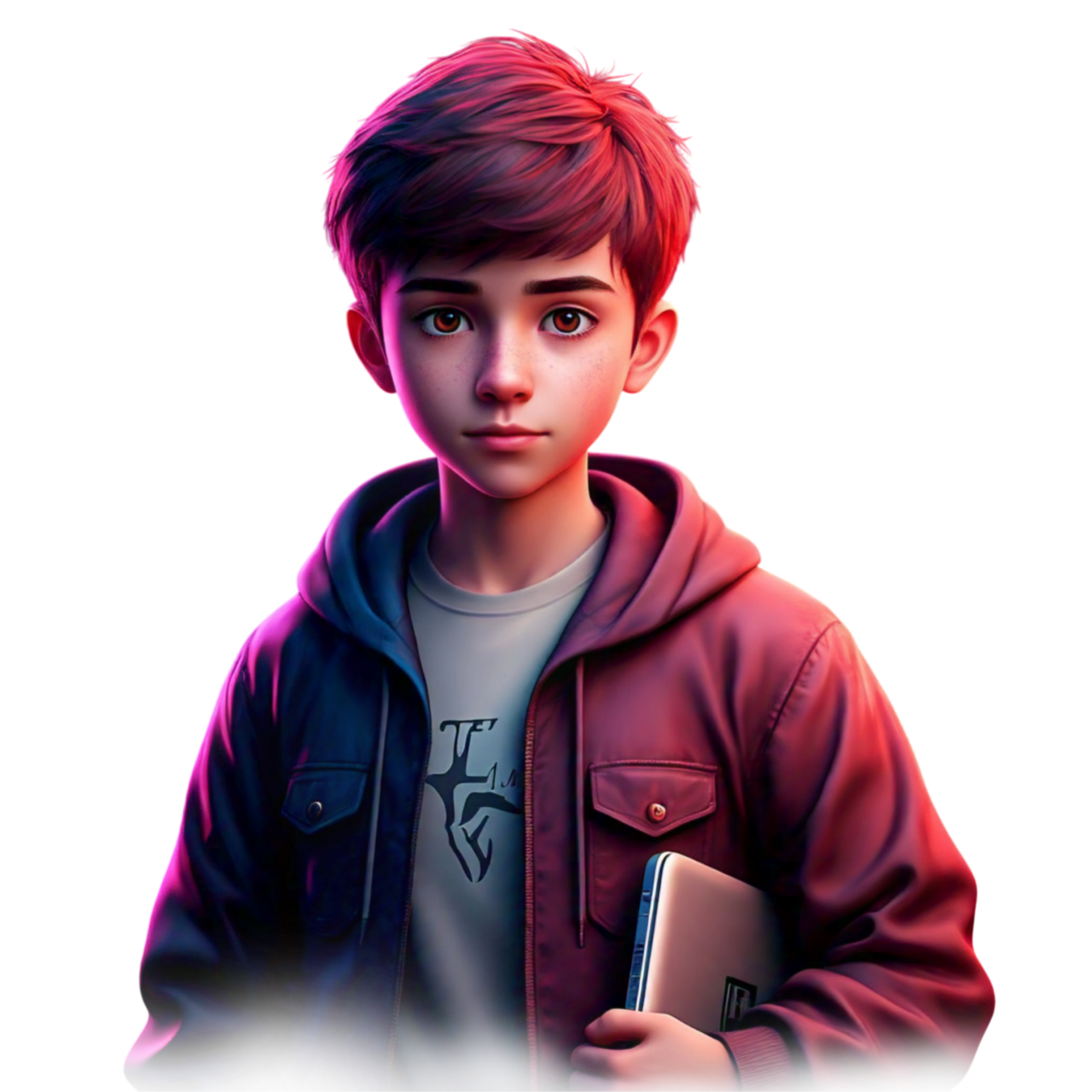
Avisek Ray
I am a skilled full-stack developer with expertise in Python (Django, FastAPI) and JavaScript (Next.js, React). With over a year of experience, I’ve delivered scalable web applications, including a news website and an AI-powered project planner. I focus on creating secure, high-performance solutions while continually expanding my skills in SQLAlchemy, Docker, and advanced Python practices. Driven by curiosity and a passion for problem-solving, I aim to build impactful, innovative applications