Master Golang in 30 Days: A Comprehensive Day-Wise Roadmap from Basics to Advanced
Looking to learn Golang from scratch and become an expert? This 30-day roadmap takes you step-by-step through the essentials, from basic syntax and control flow to advanced concepts like concurrency, WebSockets, and real-world project deployment. Perfect for beginners and those aiming to enhance their Go skills!
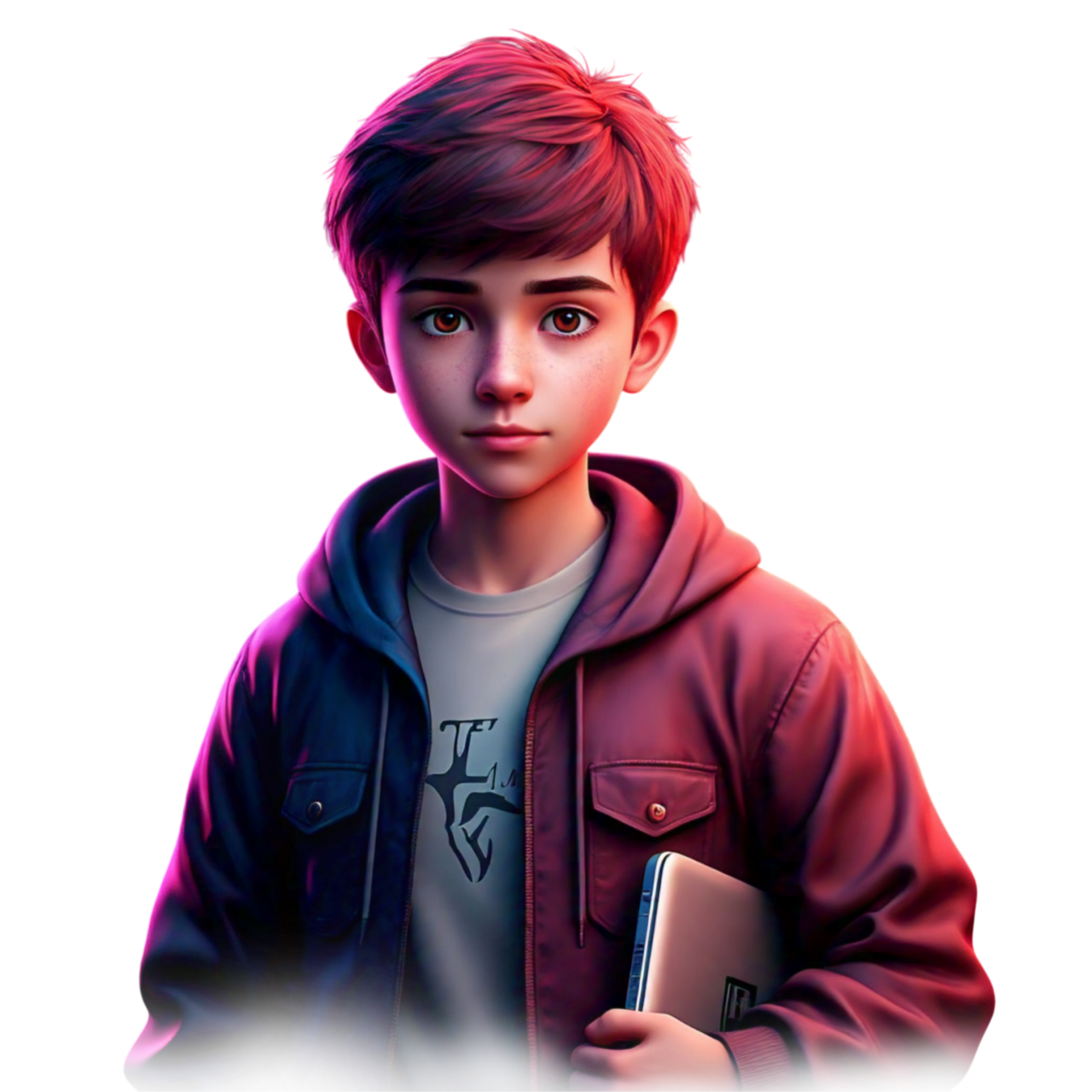
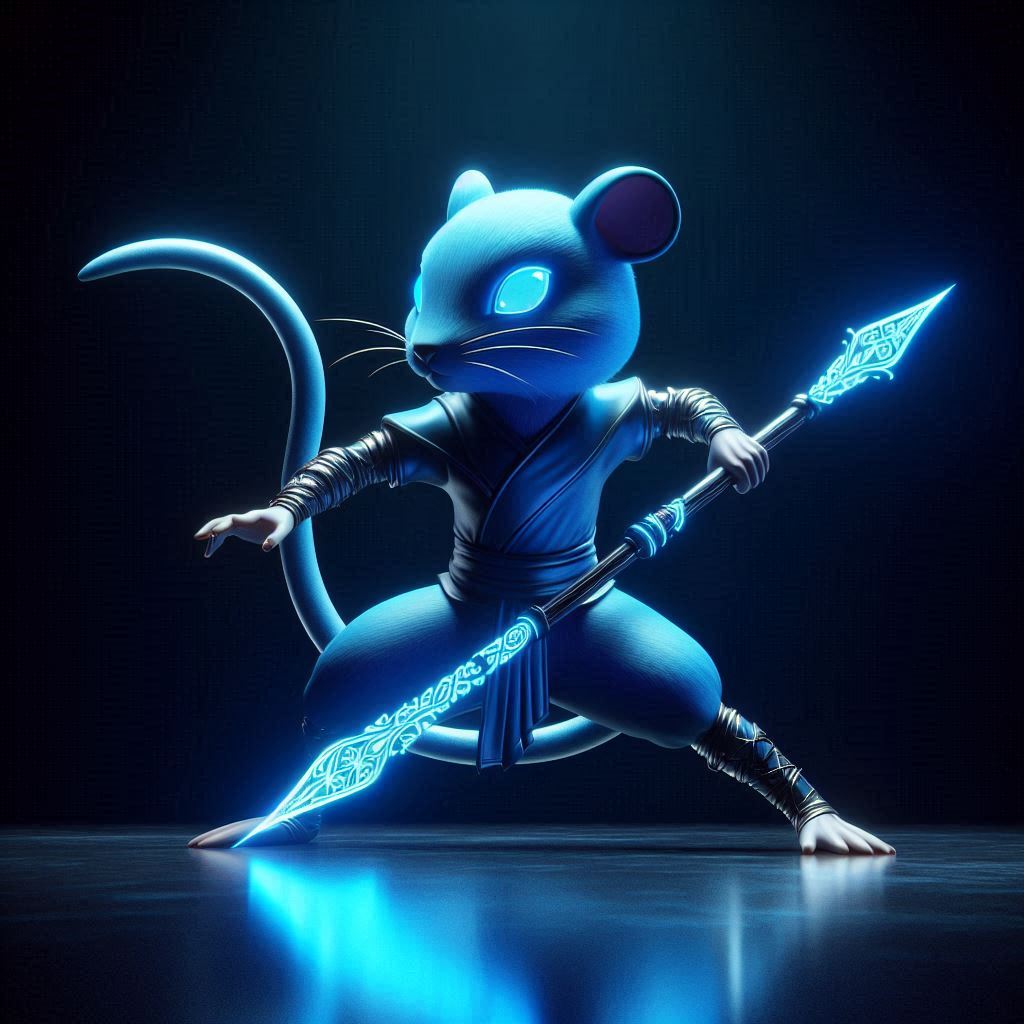
Become a Go Developer in 30 Days: The Ultimate Step-by-Step Learning Roadmap
Week 1: Basics of Go
Day 1: Introduction to Go
- What is Go? Features and use cases.
- Install Go and set up your environment.
- Write your first "Hello, World!" program.
- Learn Go syntax basics: variables, constants, and data types.
Day 2: Basic Syntax and Operators
- Learn basic operators: arithmetic, logical, and comparison.
- Input and output in Go (
fmt
package). - Basic coding exercises (calculator, temperature converter).
Day 3: Control Flow
- Conditional statements:
if
,else
,switch
. - Loops:
for
,break
,continue
. - Nested loops and patterns (simple exercises).
Day 4: Functions
- Function declaration and calling.
- Parameters and return values.
- Named return values.
- Variadic functions.
Day 5: Arrays and Slices
- Understand arrays in Go.
- Work with slices (dynamic arrays).
- Common slice operations: append, copy, and slicing.
- Practice problems with arrays and slices.
Day 6: Strings and String Manipulation
- Strings in Go: basic operations.
- String formatting and concatenation.
- Common string functions from the
strings
package. - Write a small program to count word occurrences in a string.
Day 7: Maps and Ranges
- Understand maps (key-value pairs).
- Adding, deleting, and updating map elements.
- Iterating with
range
. - Practice: Word frequency counter using maps.
Week 2: Intermediate Go
Day 8: Structs and Methods
- Understand structs and how to define them.
- Add methods to structs.
- Create constructors for structs.
Day 9: Pointers
- Basics of pointers:
&
and*
operators. - Passing by value vs. passing by reference.
- Use pointers in structs and functions.
Day 10: Interfaces
- Understand interfaces and their importance.
- Implement interfaces with structs.
- Empty interfaces and type assertion.
Day 11: Packages and Modules
- Understand Go’s package structure.
- Create and use custom packages.
- Use Go Modules (
go mod init
andgo.mod
file).
Day 12: Error Handling
- Understand error handling in Go.
- Use the
errors
package. - Custom error types and handling.
Day 13: File Handling
- Read from and write to files.
- Working with directories.
- Example: Create a program to log data to a file.
Day 14: Goroutines and Channels
- Understand Goroutines for concurrency.
- Channels for communication between Goroutines.
- Buffered vs. unbuffered channels.
Week 3: Advanced Go
Day 15: Select Statement
- Use the
select
statement to handle multiple channel operations. - Example: Implement a timeout mechanism.
Day 16: Mutex and WaitGroups
- Synchronize Goroutines with Mutex.
- Use
sync.WaitGroup
for Goroutine synchronization. - Practice: Write a simple worker pool.
Day 17: Context Package
- Use the
context
package for managing Goroutines. - Example: Graceful shutdown of Goroutines.
Day 18: Testing in Go
- Write unit tests using the
testing
package. - Table-driven tests.
- Benchmarking and examples.
Day 19: Reflection
- Learn about Go's
reflect
package. - Use cases of reflection in dynamic programming.
- Example: Inspect struct fields and methods.
Day 20: Advanced Error Handling
- Use
errors.Is
anderrors.As
for error wrapping. - Understand error propagation.
- Create custom error types with additional context.
Day 21: Logging
- Use the
log
package for basic logging. - Integrate third-party logging libraries (e.g., Logrus or Zerolog).
- Structured logging in Go.
Week 4: Building Real-World Applications
Day 22: HTTP Server with net/http
- Build a simple HTTP server.
- Handle routes and serve static files.
- Example: Create a basic REST API.
Day 23: Middleware in Go
- Understand middleware in Go HTTP servers.
- Write custom middleware for logging and authentication.
- Practice: Add middleware to the REST API.
Day 24: Go with Databases
- Connect to a database using
database/sql
. - Perform CRUD operations.
- Use GORM (Go ORM) for advanced database management.
Day 25: JSON and APIs
- Parse and generate JSON with the
encoding/json
package. - Example: Consume a third-party API.
Day 26: WebSockets in Go
- Build a WebSocket server with
gorilla/websocket
. - Example: Real-time chat application.
Day 27: Dependency Injection
- Understand dependency injection principles.
- Implement DI in your Go application.
Day 28: Build and Deploy
- Compile and build Go binaries.
- Use
go build
,go run
, andgo install
. - Example: Deploy a Go application using Docker.
Day 29: Go Modules and Versioning
- Advanced Go Modules usage.
- Manage dependencies and versioning effectively.
Day 30: Final Project
- Combine all your knowledge into a real-world project:
- A complete REST API with CRUD, middleware, authentication, and database support.
- Add logging, error handling, and deploy it using Docker.
Tips for Learning:
- Practice every day with real-world examples.
- Use online Go playgrounds (e.g., play.golang.org) for quick experiments.
- Refer to the official Go documentation: https://golang.org/doc/
- Contribute to open-source projects to deepen your understanding.
About the Author
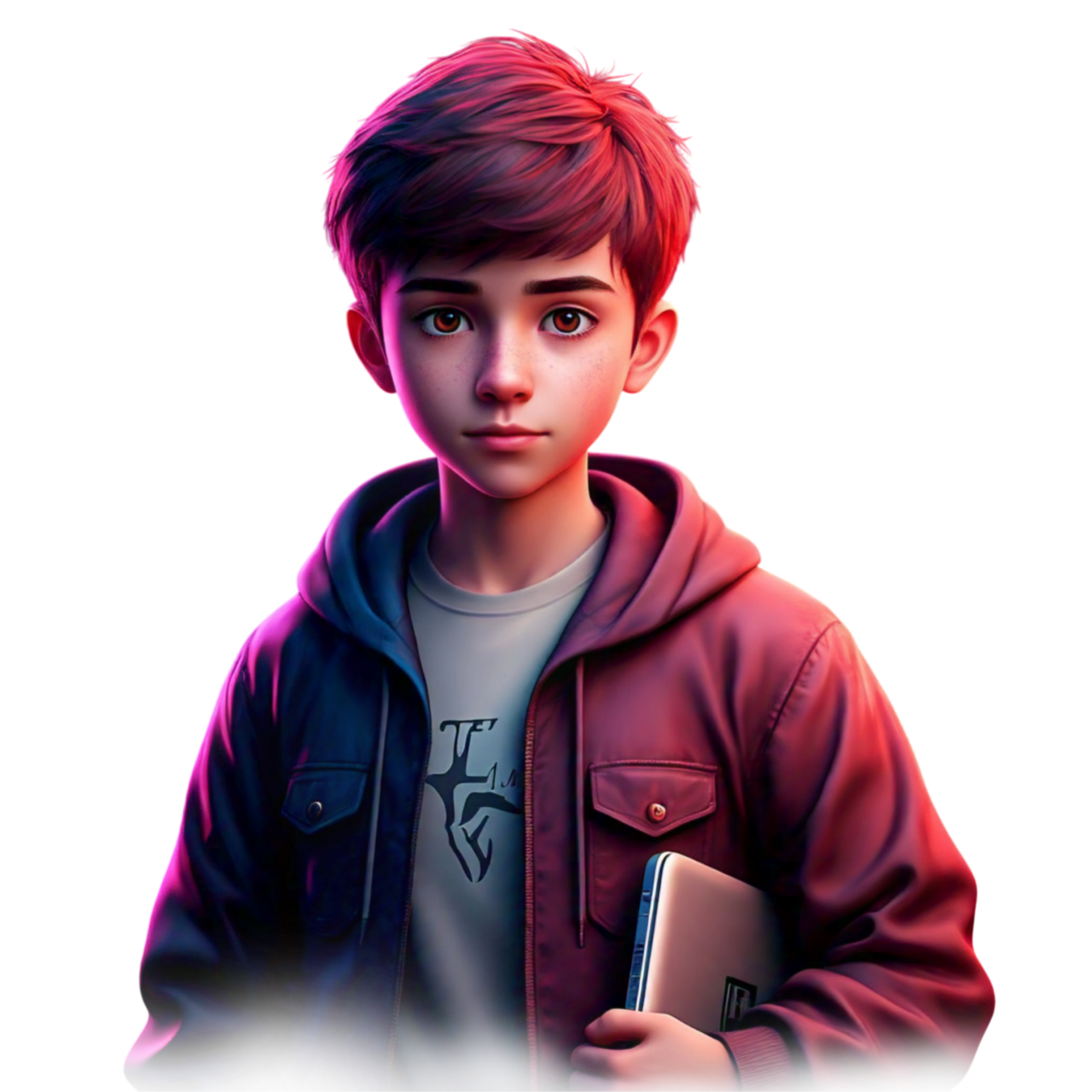
Avisek Ray
I am a skilled full-stack developer with expertise in Python (Django, FastAPI) and JavaScript (Next.js, React). With over a year of experience, I’ve delivered scalable web applications, including a news website and an AI-powered project planner. I focus on creating secure, high-performance solutions while continually expanding my skills in SQLAlchemy, Docker, and advanced Python practices. Driven by curiosity and a passion for problem-solving, I aim to build impactful, innovative applications